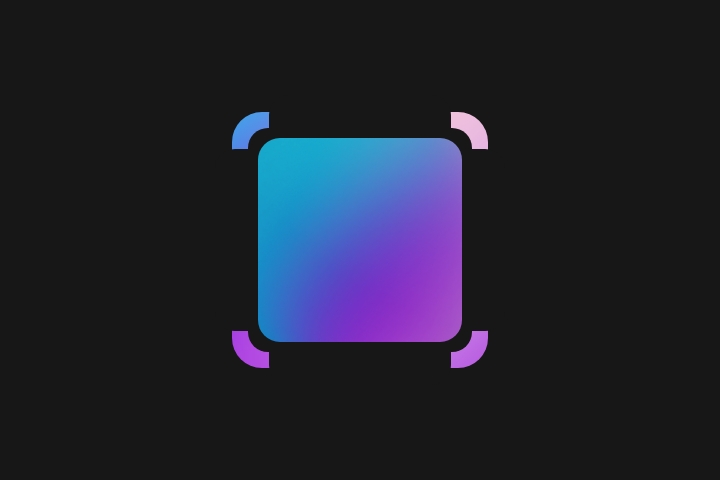
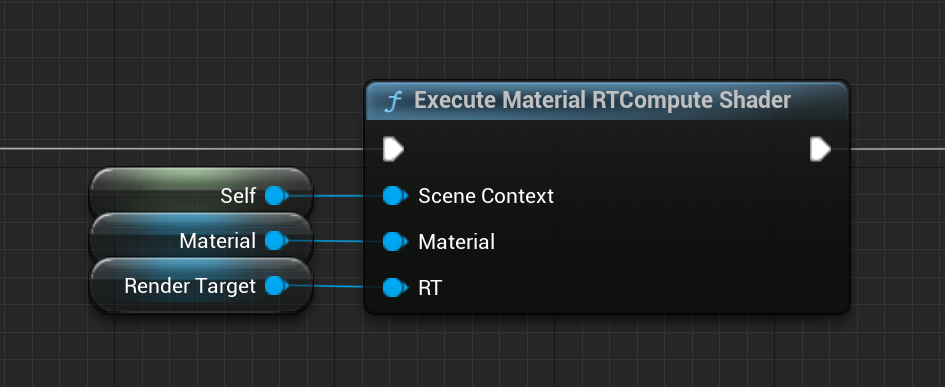
Material graph to render target via compute in UE5
This is similar to the Draw Material to Render Target
node provided by Unreal but executed through compute.
Get the code
Usage
- Generate or download the template using the instructions above
- Rebuild your project
- Create or navigate to an existing Blueprint
- Right-click and add the node called “Execute Material RTCompute Shader”
Notes
- The render target you use should be a multiple of 32. If not the sampler might go over the edge and wrap back around (depending on your settings).
Helpful reading
Sample snippet
// rtmat.usf
#include "/Engine/Generated/Material.ush"
#include "/Engine/Public/Platform.ush"
RWTexture2D<float3> RenderTarget;
[numthreads(THREADS_X, THREADS_Y, THREADS_Z)]
void RTMatExample(
uint3 DispatchThreadId : SV_DispatchThreadID,
uint GroupIndex : SV_GroupIndex )
{
float4 SvPosition = float4(DispatchThreadId.xy, 0, 0);
FMaterialPixelParameters MaterialParameters = MakeInitializedMaterialPixelParameters();
// There are various inputs we can provide to the material graph via FMaterialPixelParameters
// See: https://github.com/EpicGames/UnrealEngine/blob/release/Engine/Shaders/Private/MaterialTemplate.ush#L262
MaterialParameters.VertexColor = half4(1, 1, 0, 1);
FPixelMaterialInputs PixelMaterialInputs = (FPixelMaterialInputs)0;
// This is the call to the material graph
CalcMaterialParameters(MaterialParameters, PixelMaterialInputs, SvPosition, true);
// PixelMaterialInputs is a struct that contains the outputs of the material graph
// Use the provided helper methods ( GetMaterialXYZ(...) ) to access the outputs
// See: https://github.com/chendi-YU/UnrealEngine/blob/master/Engine/Shaders/MaterialTemplate.usf#L1298
RenderTarget[DispatchThreadId.xy] = GetMaterialBaseColor(PixelMaterialInputs);
}