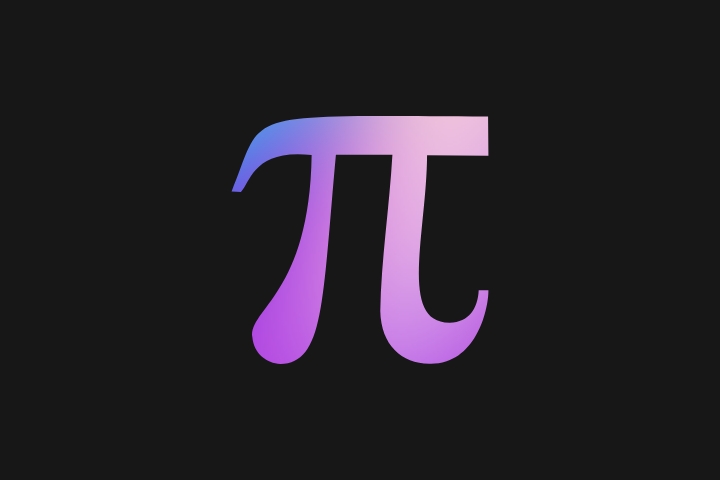
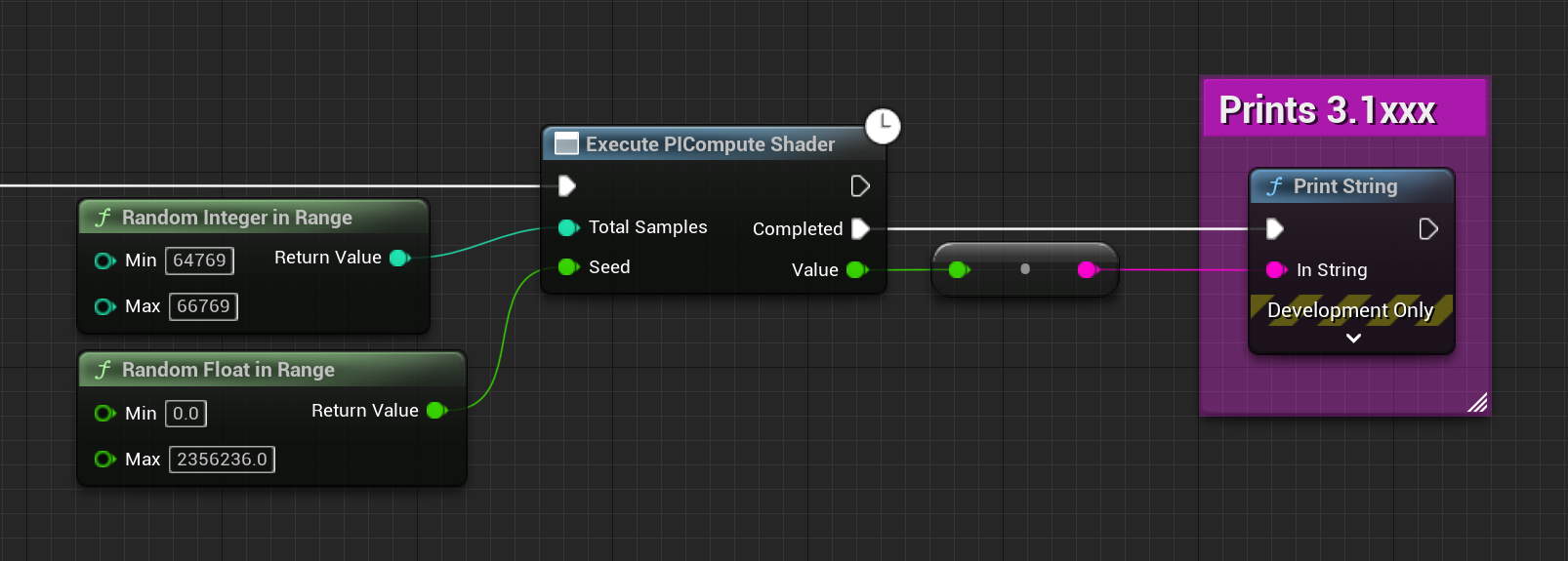
Calculate PI via compute in UE5
This example approximates PI in parallel using the Monte Carlo method. When running you’re only going to be able to get about 2-3 decimal places of accuracy.
Get the code
Usage
- Generate or download the template using the instructions above
- Rebuild your project
- Create or navigate to an existing Blueprint
- Right-click and add the node called “Execute PICompute Shader”
Notes
- The mechanism used for detecting when the results are ready on the CPU is for example purposes (see
F[NAME]Interface::DispatchRenderThread - RunnerFunc
). A better strategy would be to queue and check a list of buffers per tick in an external singleton.
Helpful reading
- Using Compute Shaders in Unreal Engine 4
- Overview of Shaders in Plugins
- UE4ShaderPluginDemo
- Render Dependency Graph
Sample snippet
// pi.usf
#include "/Engine/Public/Platform.ush"
// Calculate π using the monte carlo method
// https://en.wikipedia.org/wiki/Monte_Carlo_method
// Output has 1 element: [numInCircle]
RWBuffer<uint> Output;
// Seed the random number generator
float Seed = 0.0f;
float random( float2 p )
{
float2 r = float2(
23.14069263277926,
2.665144142690225
);
return frac( cos( dot(p,r) ) * 12345.6789 );
}
[numthreads(THREADS_X, THREADS_Y, THREADS_Z)]
void PIExample(
uint3 DispatchThreadId : SV_DispatchThreadID,
uint GroupIndex : SV_GroupIndex )
{
float2 RandomPosition = float2(
random(float2(GroupIndex * THREADS_X + DispatchThreadId.x, 10 + Seed)),
random(float2(GroupIndex * THREADS_X + DispatchThreadId.x, 20 * Seed))
);
float Dist = length(RandomPosition);
if (Dist < 1.0f) {
uint Dummy;
InterlockedAdd(Output[0], 1, Dummy);
}
}