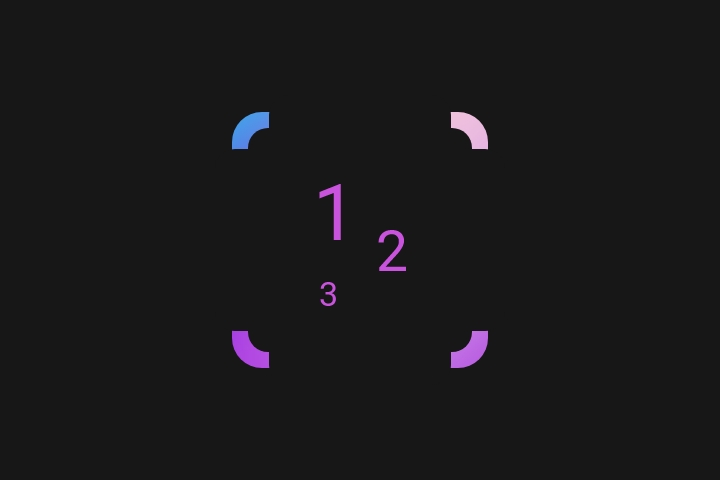
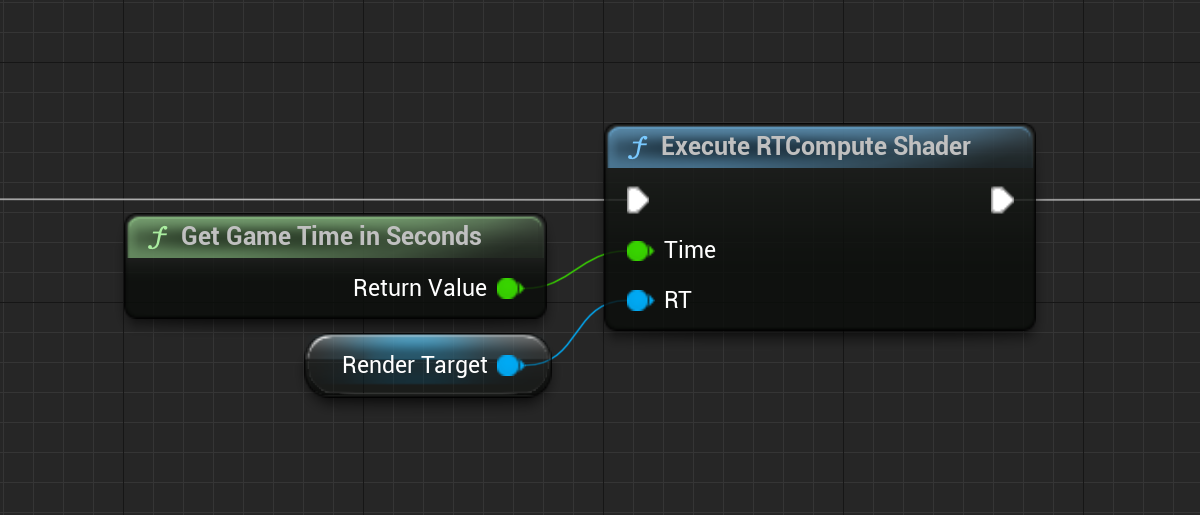
Writing to a render target via compute in UE5
Drawing to render targets from compute shaders is an extremely useful method to render all sorts of things that need multiple passes or expensive calculations cached.
Get the code
Applications
- 2d simulations (water, game of life, etc)
- Complex/procedural texture baking
- Custom rendering that doesn’t need to be run every frame
Usage
- Generate or download the template using the instructions above
- Rebuild your project
- Create or navigate to an existing Blueprint
- Right-click and add the node called “Execute RTCompute Shader”
Notes
- The render target you use should be a multiple of 32. If not the sampler might go over the edge and wrap back around (depending on your settings).
Helpful reading
Sample snippet
// rt.usf
#include "/Engine/Generated/Material.ush"
#include "/Engine/Public/Platform.ush"
RWTexture2D<float3> RenderTarget;
[numthreads(THREADS_X, THREADS_Y, THREADS_Z)]
void RTExample(
uint3 DispatchThreadId : SV_DispatchThreadID,
uint GroupIndex : SV_GroupIndex )
{
// Simple checkerboard
int x = floor(DispatchThreadId.x / 16.f);
int y = floor(DispatchThreadId.y / 16.f);
int c = (x + y % 2) % 2;
RenderTarget[DispatchThreadId.xy] = float3(c, c, c);
}